Share this
AndroidX Biometric Overview
by Seven Peaks on Apr 18, 2022 10:35:00 AM
Written by our experienced Android Developer
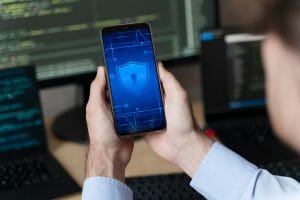
Introduction
Biometric authentication in mobile devices has been around for quite a few years now and has been adopted largely by device manufacturers and also by users. It is highly likely that every smartphone user has already had some experience with biometric authentication. It is widely used mainly by financial applications to ensure users’ convenience and safety.
In this post, I would like to describe as a developer how we can easily integrate these powerful AndroidX Biometric APIs in our Android Applications and get full advantage of them.
A bit on Background
FingerPrintManager class was introduced in Android 6.0 (API level 23) which would coordinate access to only fingerprint hardware. However, It did not have any UI so developers had to implement customized, device-specific implementations for biometric authentication. Developing customized UI was never easy, on top of that, in-display sensors made life more difficult.
After receiving feedback from developers, Android 9 (API level 28) introduced a standardized fingerprint UI policy. In addition, BiometricPrompt was introduced to onboard more sensors than just fingerprints. This not only provides developers with a set of APIs that they can customize effortlessly to show consistent and familiar UI to the user but also hide the complexity of communicating a wide range of biometric hardware available in the devices. This also benefited OEMs as they now can customize these APIs to implement their own style and also introduce new biometrics without having to worry about developers’ adaptability.
In Android 10 (API level 29) Android Framework and Security team enhanced AndroidX Biometric Library even more which has made all of the biometric behavior from Android 10 available to all devices that run Android 6.0 (API level 23) or higher. In addition to supporting multiple biometric authentications, they introduced BiometricManager which enables developers to check whether a device supports biometric authentication with a single API.
Backward Compatibility
As mentioned earlier, AndroidX Biometric was introduced in Android 10 and it provides support for biometric authentication all the way back to API 23 and to only access device credentials like password, pin, and patterns, it extends support from API 21.
Integration:
Now the fun part!
Biometric authentication can be integrated with 5 simple steps:
Step 1: Add the Gradle dependency
Add the following dependency in the app-level Gradle file
implementation "androidx.biometric:biometric:1.1.0"
Step 2: Check whether the device supports biometric authentication
Biometric library provides BiometricManager to check if the user’s device supports biometric features before you perform any authentication. If it does not, you can show a user-friendly message stating the device does not support biometric authentication.
biometricManager.canAuthenticate(int)
The int value represents the type of Authentication you want. There are 3 types of Authenticators you can choose from:
- BIOMETRIC_WEAK for non-crypto authentication
- BIOMETRIC_STRONG for crypto-based authentication
- DEVICE_CREDENTIAL for non-biometric Credential
private fun authenticateUser() {
val biometricManager = BiometricManager.from(this)
when (biometricManager.canAuthenticate(BIOMETRIC_WEAK)) {
BiometricManager.BIOMETRIC_SUCCESS ->
showBiometricPrompt()
BiometricManager.BIOMETRIC_ERROR_NO_HARDWARE ->
showMessage("There is no suitable hardware")
BiometricManager.BIOMETRIC_ERROR_HW_UNAVAILABLE ->
showMessage("The hardware is unavailable. Try again later")
BiometricManager.BIOMETRIC_ERROR_NONE_ENROLLED ->
showMessage("No biometric or device credential is enrolled")
BiometricManager.BIOMETRIC_ERROR_SECURITY_UPDATE_REQUIRED ->
showMessage("A security vulnerability has been discovered with one or more hardware sensors")
BiometricManager.BIOMETRIC_ERROR_UNSUPPORTED ->
showMessage("The specified options are incompatible with the current Android version")
BiometricManager.BIOMETRIC_STATUS_UNKNOWN ->
showMessage("Unable to determine whether the user can authenticate")
}
}
We can combine these Authenticators like BIOMETRIC_WEAK | DEVICE_CREDENTIAL
But not all the combination is not supported across all the APIs, for example, DEVICE_CREDENTIAL alone is unsupported prior to API 30 BIOMETRIC_STRONG | DEVICE_CREDENTIAL is unsupported on API 28–29
Step 3: Build the UI with PromptInfo object
PromptInfo object contains the metadata and configurations for our BiometricPrompt. We can customize our prompt via the methods setTitle, setSubtitle, setDescription, and setNegativeButtonText. With setAllowedAuthenticators, we can specify if we want to allow device credentials (PIN, pattern or password) as a fallback, and through setConfirmationRequired, we can enable or disable explicit confirmation for implicit authentication methods (like face and iris). It could be useful for scenarios like making a fund transaction where you want to reconfirm with the user before initiating the process.
val promptInfo = BiometricPrompt.PromptInfo.Builder()
.setTitle("AndroidX Biometric")
.setSubtitle("Authenticate user via Biometric")
.setDescription("Please authenticate yourself here")
.setAllowedAuthenticators(BIOMETRIC_WEAK)
.setNegativeButtonText("Cancel")
.setConfirmationRequired(true)
.build()
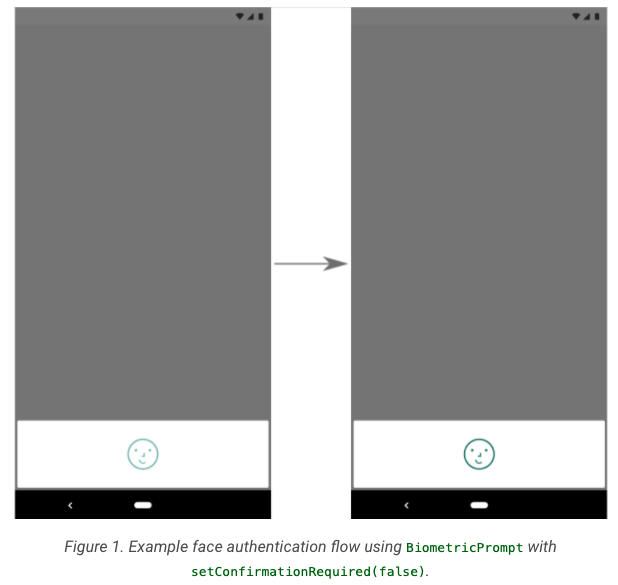
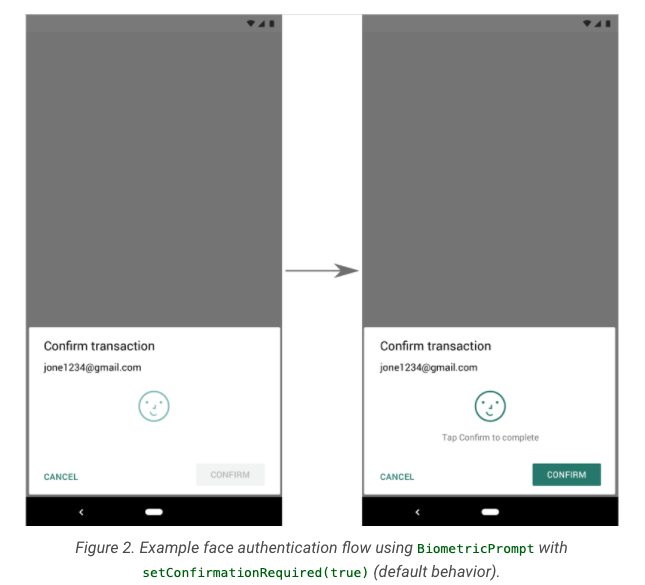
However, showing this explicit confirmation depends on the devices, as a result, explicit confirmation could be ignored in some devices even though it was set to true via the setConfirmationRequired method.
Step 4: Create an instance of BiometricPrompt
AndroidX BiometricPrompt does all the heavy lifting for you. It takes the UI metadata, shows the authentication prompt to the user, and returns the authentication result as a callback. It needs 4 components to work:
- An Activity or Fragment to provide the fragmentManager which will be used to show the authentication dialog.
- The PromptInfo object which contains the UI metadata
- An executor that defines on which thread this callback runs. If we want to run our callback on the UI thread, we can use the main executor from the ContextCompat.getMainExecutor() method.
- An AuthenticationCallback through which we get our authentication result. The AuthenticationCallback contains 3 methods that we can override: onAuthenticationSucceeded, which will be called in case of successful authentication, onAuthenticationFailed, which is called when a biometric was not recognized (for example because a wrong finger was put on the fingerprint sensor), and onAuthenticationError, which is triggered in case of an unrecoverable error, such as when the user canceled the authentication process or when there is no biometrics enrolled on the device. Unlike, onAuthenticationSucceeded and onAuthenticationError, onAuthenticationFailed is not a terminal callback, which means you can expect to receive multiple callbacks in this method.
private val authCallback = object : BiometricPrompt.AuthenticationCallback() {
override fun onAuthenticationSucceeded(result: BiometricPrompt.AuthenticationResult) {
showMessage("Authentication successful")
}
override fun onAuthenticationError(errorCode: Int, errString: CharSequence) {
showMessage("Unrecoverable error => $errString")
}
override fun onAuthenticationFailed() {
showMessage("Could not recognise the user")
}
}
// Step 4
val biometricPrompt = BiometricPrompt(this@MainActivity, ContextCompat.getMainExecutor(this), authCallback)
Step 5: Ask the user to authenticate
Finally, show the biometric prompt to users and ask them to authenticate.biometricPrompt.authenticate(promptInfo)
This prompt can be canceled viabiometricPrompt.cancelAuthentication()
If your app requires the user to authenticate using a Strong biometric or needs to perform cryptographic operations in KeyStore, you should use authenticate(PromptInfo, CryptoObject)
instead.
Final Thoughts:
The power of AndroidX Biometric Library lies in, how it has abstracted all the complexities of communicating with different kinds of biometric authentication sensors (like fingerprint, face and iris) and provided simple APIs to developers and OEMs to customize. However, as of now, there is no way to detect which biometric sensor a device has via this library. Hence, you might need to show messages to the user with a generic text like Biometric rather than showing a more specific one like Fingerprint, Face Id.
I hope I have helped you to learn something new today. Happy learning!
Written By
Sifat UI Haque, Android Tech Lead @ Seven Peaks Software
Click here to follow Sifat on Medium!
Share this
- FinTech (13)
- Career (12)
- Expert Spotlight (12)
- Thought Leadership (11)
- Product Growth (9)
- Software Development (9)
- Data and Analytics (7)
- Product Design (7)
- Digital Product (6)
- AI (5)
- Cloud (5)
- Data (5)
- Design Thinking (5)
- InsurTech (5)
- QA (5)
- Agile (4)
- CSR (4)
- Company (4)
- Digital Transformation (4)
- Financial Inclusion (4)
- JavaScript (4)
- Seven Peaks Insights (4)
- Trend (4)
- UX Design (4)
- UX Research (4)
- .NET (3)
- Android Developer (3)
- Android Development (3)
- Azure (3)
- Banking (3)
- DevOps (3)
- IoT (3)
- Product-Centric Mindset (3)
- Service Design (3)
- CDP (2)
- Cloud Development (2)
- Customer Data Platform (2)
- E-wallet (2)
- Expat (2)
- Hybrid App (2)
- Kotlin (2)
- Product Owner (2)
- Software Tester (2)
- SwiftUI (2)
- UI (2)
- UX (2)
- UX Writing (2)
- Visual Design (2)
- iOS Development (2)
- .NET 8 (1)
- 2023 (1)
- 4IR (1)
- 5G (1)
- API (1)
- Agritech (1)
- AndroidX Biometric (1)
- App Development (1)
- Azure OpenAI Service (1)
- Backend (1)
- Brand Loyalty (1)
- CI/CD (1)
- Conversions (1)
- Cross-Platform Application (1)
- Dashboard (1)
- Digital (1)
- Digital Healthcare (1)
- Digital ID (1)
- Digital Landscape (1)
- Engineer (1)
- Expert Interview (1)
- Fiddler (1)
- Figma (1)
- Financial Times (1)
- GraphQL (1)
- Hilt (1)
- IT outsourcing (1)
- KYC (1)
- MVP (1)
- MVVM (1)
- Metaverse (1)
- Morphosis (1)
- Native App (1)
- New C# (1)
- Newsletter (1)
- Node.js (1)
- Payment (1)
- Platform Engineer (1)
- Platform Engineering Jobs (1)
- Platform Engineering Services (1)
- Product Discovery (1)
- Project Manager (1)
- Rabbit MQ (1)
- React (1)
- ReactJS (1)
- Stripe (1)
- Super App (1)
- Turnkey (1)
- UIkit (1)
- UX Strategy (1)
- Web 3.0 (1)
- Web-Debugging Tool (1)
- June 2025 (10)
- May 2025 (2)
- April 2025 (2)
- March 2025 (4)
- February 2025 (1)
- January 2025 (3)
- December 2024 (4)
- November 2024 (2)
- September 2024 (4)
- August 2024 (3)
- July 2024 (6)
- April 2024 (1)
- March 2024 (7)
- February 2024 (14)
- January 2024 (13)
- December 2023 (9)
- November 2023 (9)
- October 2023 (2)
- September 2023 (6)
- August 2023 (6)
- June 2023 (4)
- May 2023 (4)
- April 2023 (1)
- March 2023 (1)
- November 2022 (1)
- August 2022 (4)
- July 2022 (1)
- June 2022 (6)
- April 2022 (6)
- March 2022 (4)
- February 2022 (8)
- January 2022 (4)
- December 2021 (1)
- November 2021 (2)
- October 2021 (2)
- September 2021 (1)
- August 2021 (3)
- July 2021 (1)
- June 2021 (2)
- May 2021 (1)
- March 2021 (4)
- February 2021 (5)
- December 2020 (4)
- November 2020 (1)
- June 2020 (1)
- April 2020 (1)